题目:有1、2、3、4个数字,能组成多少个互不相同且无重复数字的三位数?都是多少?
import java.util.*; // 题目:有1、2、3、4个数字,能组成多少个互不相同且无重复数字的三位数?都是多少? public class Text1 { @SuppressWarnings({ "rawtypes", "unchecked" }) public static void main(String[] args) { // 方法一 /* int a[]={1,2,3,4}; int n; int count=0; Vector v = new Vector(); for(int i=1;i<=4;i++){ for(int j=1;j<=4;j++){ if(i!=j){ for(int k=1;k<=4;k++){ if(i!=k&&j!=k){ n=i*100+j*10+k; v.add(n); count++; } } } } } System.out.println(count); System.out.println(v); */ // 方法二 int[] s={1,2,3,4}; int a=0; for(int i=0;i<s.length;i++){ for(int k=0;k<s.length;k++){ for(int j=0;j<s.length;j++){ if(i != k && k != j && i != j){ System.out.println((s[i]*100)+(s[k]*10)+s[j]); a++; System.out.println("第"+a+"种"); } } } } } }
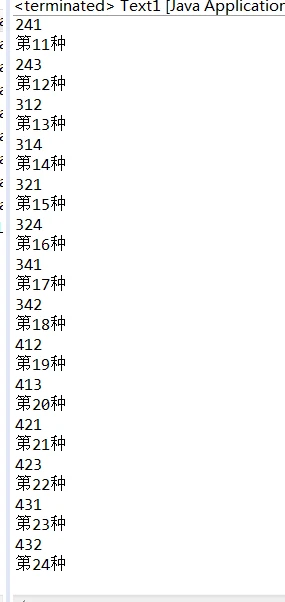
题目:有一分数序列:2/1,3/2,5/3,8/5,13/8,21/13...求出这个数列的前20项之和
import java.util.ArrayList; import java.util.List; //题目:有一分数序列:2/1,3/2,5/3,8/5,13/8,21/13...求出这个数列的前20项之和 //分子:2 3 5 8 13 21······ 前一个数的分子与分母之和 //分母:1 2 3 5 8 13······ 前一个数的分子 public class Text2 { public static void main(String[]args){ // 方法一 float b = 2; float a = 1; float sum = 0; for(int i = 0;i < 20;i++){ if(i%5 == 0){ System.out.println(); System.out.print(b+"/"+a+"+"); float temp = 0; sum += b/a; temp = a; a = b; b = temp + b; }else if(i == 19){ System.out.print(b+"/"+a+"="); JAVA方法基础例题 float temp = 0; sum += b/a; temp = a; a = b; b = temp + b; }else{ System.out.print(b+"/"+a+"+"); float temp = 0; sum += b/a; temp = a; a = b; b = temp + b; } } System.out.println(sum); // 方法二 /* double a = 2; double b = 1; double sum = 0; List<Object> li = new ArrayList<Object>(); for(int i = 0;i<20;i++){ li.add(i,a/b); if(a%b != 0){ System.out.println(a+"/"+b); } sum += a/b; double c =a; a = a+b; b=c; System.out.println(sum); } */ // 方法三 /* float s=1; //分子 float x=1; //分母 float sum=0; float temp=0; for(int i=0;i<20;i++){ temp = x; x=s; s = s+temp; sum += s/x; } System.out.println("前20项之和为:"+sum);*/ } }
题目:求1!+2!+3!+...+20!的和(5!表示5的阶乘, 即5*4*3*2*1)
//题目:求1!+2!+3!+...+20!的和(5!表示5的阶乘, 即5*4*3*2*1) public class Text3 { public static void main(String[] args){ //方法一 /* long sum = 0; long n=1; for(int i=1;i<=20;i++){ n *=i; sum +=n; System.out.println(n); } System.out.println("1!+2!+3!+...+20!的和: "+sum); */ // 方法二 System.out.println(fact(20)); long a = 0; for(int i=1;i<21;i++){ a += fact(i); } System.out.println(a); } private static long fact(long i) { if(i==1){ return 1; }else{ return fact(i-1)*i; } } }
题目:一个5位数,判断它是不是回文数。即12321是回文数,个位与万位相同,十位与千位相同。
import java.util.Scanner; // 题目:一个5位数,判断它是不是回文数。即12321是回文数,个位与万位相同,十位与千位相同。 // public class Text4 { public static void main(String[] args) { //方法一 /* Scanner in = new Scanner(System.in); String num = in.next(); if (num.matches("\d+")) { char[] nums = num.toCharArray(); String num1=""; for (int i = 0; i < nums.length; i++) { num1+=nums[i]; } if(num1.equals(nums)){ System.out.println("是回文数"); }else{ System.out.println("不是回文数"); } }*/ //方法二 Scanner s = new Scanner(System.in); int a = s.nextInt(); if (a / 10000 == a % 10 && (a % 10000) / 1000 == (a % 100) / 10) { System.out.println("这是一个回文数"); } else { System.out.println("这不是一个回文数了"); } s.close(); //方法三 //Scanner sc = new Scanner(System.in); // String s = sc.nextLine(); // String[] ss = s.split(""); // if (ss[0].equals(ss[4]) && ss[1].equals(ss[3])) { // System.out.println("是回文数"); // } else { // System.out.println("不是回文数"); // } // sc.close(); } }
题目:先写一个程序, 随机生成一个3*3的矩阵数字(1-9数字全部用到不能重复), 然后求这个3*3矩阵对角线元素之和
import java.util.ArrayList; import java.util.Collections; import java.util.List; import java.util.Random; //题目:先写一个程序, 随机生成一个3*3的矩阵数字(1-9数字全部用到不能重复), 然后求这个3*3矩阵对角线元素之和 public class Text5 { @SuppressWarnings({ "rawtypes", "unchecked" }) public static void main(String[] args){ List<Integer> l = new ArrayList<Integer>();// 不能重复所以用集合可以把已经赋值的删除 for (int i = 1; i < 10; i++) { l.add(i - 1, i); } Collections.shuffle(l); sunji(l); List l1 = new ArrayList(); List l2 = new ArrayList(); List l3 = new ArrayList(); Random r = new Random(); List list = new ArrayList(); for (int i = 1; i <= 9; i++) { list.add(i); } for (int j = 0; j < 3; j++) { l1.add(list.remove(r.nextInt(9 - j))); } for (int j = 0; j < 3; j++) { l2.add(list.remove(r.nextInt(6 - j))); } for (int j = 0; j < 3; j++) { l3.add(list.remove(r.nextInt(3 - j))); } System.out.println(l1); System.out.println(l2); System.out.println(l3); System.out.println((int) l1.get(0) + (int) l2.get(1) + (int) l3.get(2) + (int) l1.get(2) + (int) l3.get(0)); } private static void sunji(List<Integer> l) { int t[][] = new int[3][3]; Random r = new Random(); for (int a = 0; a < 3; a++) { for (int b = 0; b < 3; b++) { int c = r.nextInt(l.size());// 用一个变量来接收随机数,随机数的最大数不能大于集合的长度, t[a][b] = l.get(c);// 从集合中取到的数赋给数组 l.remove(c);// 赋值以后再把这个数从集合中删除 } } int sum1 = t[0][2] + t[1][1] + t[2][0];// 计算对角线上的数的和 int sum2 = t[0][0] + t[1][1] + t[2][2]; for (int x = 0; x < 3; x++) { for (int y = 0; y < 3; y++) { System.out.println(t[x][y]); } } System.out.println(sum1 + " " + sum2); } public static boolean check(List<Integer> list, int n) { if (list.contains(n)) { return false; } return true; } }
回忆包装类对应的基础数据类型和基本方法的使用 将字符串转成数字(Long, Integer, Short, Byte, Double, Float) 字符串转换成字符数组, 并分别输出
import java.util.Arrays; // 回忆包装类对应的基础数据类型和基本方法的使用 //将字符串转成数字(Long, Integer, Short, Byte, Double, Float) //字符串转换成字符数组, 并分别输出 public class Text6 { public static void main(String[] args) { String s = "abcgfh"; System.out.println(Arrays.toString(s.toCharArray())); // 7 String s2 = ""; String s8 = "12365"; String s16 = "1a23c"; System.out.println(Integer.parseInt(s2, 2)); System.out.println(Integer.parseInt(s8, 8)); System.out.println(Integer.parseInt(s16, 16)); } }
取一个随便的字符串中字母出现的次数, 打印出来
import java.util.HashMap; import java.util.Map; //取一个随便的字符串中字母出现的次数, 打印出来 public class Text8 { public static void main(String[] args){ String s = "aswdddffggbzxas"; Map<String,Integer> map = new HashMap<String,Integer>(); String[] a = s.split(""); for(String ss:a){ map.put(ss,map.get(ss)==null?1:map.get(ss)+1); } System.out.println(map); } }
有一个字符串形式的任意日期是"yyyy-MM-dd"的格式, 计算这个日期到1949年10月1日差了多少天
import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; //有一个字符串形式的任意日期是"yyyy-MM-dd"的格式, 计算这个日期到1949年10月1日差了多少天 public class Text9 { public static void main(String[] args){ String s_date1 = "2017-8-4" ; String s_date2 = "1949-10-1" ; try { Date date1 = new SimpleDateFormat("yyyy-MM-dd").parse(s_date1); Date date2 = new SimpleDateFormat("yyyy-MM-dd").parse(s_date2); long m=date1.getTime()-date2.getTime(); long day=m/1000/60/60/24; System.out.println(day); } catch (ParseException e) { e.printStackTrace(); } } }
从data.txt文件中读取数据到程序中, 实现一个联动功能, 即输入主食会显示"1---馒头 2---煎饼 3---米饭", 再次输入会显示下一级菜单 data.txt文件中每一行都有被 "," 分割的三个值, 第一个值代表这项食物的编号(对于整个数据来说是唯一的), 第三个值表示所属的上一级食物分类
Food类
public class Food { private String id; private String name; private String parentid; public Food(String id, String name, String parentid) { super(); this.id = id; this.name = name; this.parentid = parentid; } public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getParentid() { return parentid; } public void setParentid(String parentid) { this.parentid = parentid; } @Override public String toString() { return "Food [id=" + id + ", name=" + name + ", parentid=" + parentid + "]"; } }
import java.io.BufferedReader; import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.io.Reader; import java.util.ArrayList; import java.util.List; import java.util.Scanner; import text3.Food; //从data.txt文件中读取数据到程序中, 实现一个联动功能, 即输入主食会显示"1---馒头 2---煎饼 3---米饭", 再次输入会显示下一级菜单 //data.txt文件中每一行都有被 "," 分割的三个值, 第一个值代表这项食物的编号(对于整个数据来说是唯一的), 第三个值表示所属的上一级食物分类 public class Text1 { public static void main(String[] args) { File file = new File("F:\java\课后作业\0803\data.txt"); List<Food> list = new ArrayList<Food>(); Reader reader = null; try { reader = new FileReader(file); BufferedReader br = new BufferedReader(reader); String s = null; while((s = br.readLine())!=null) { String[] ss = s.split(","); if(ss.length==3) { Food food = new Food(ss[0],ss[1],ss[2]); list.add(food); } } br.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } for(Food ff : list) { if("0".equals(ff.getParentid())) { System.out.println(ff.getId()+"---"+ff.getName()); } } Scanner scanner = new Scanner(System.in); boolean flag = true; String in_str = scanner.nextLine(); while(flag) { if("exit".equals(in_str)) { flag = false; } else { printFood(list, in_str); in_str = scanner.nextLine(); } } scanner.close(); } public static void printFood(List<Food> list, String pid) { for(Food ff : list) { if(pid.equals(ff.getParentid())) { System.out.println(ff.getId()+"---"+ff.getName()); } } } }
写一个带线程同步的程序模拟5个人在火车站买票, 总共有4张票(对比着课上取钱的例子来)
import javax.xml.soap.Text; //写一个带线程同步的程序模拟5个人在火车站买票, 总共有4张票(对比着课上取钱的例子来) public class Text3 { /* public int ticket =4; public void getTicket(String name){ synchronized(this){ if(ticket>0){ ticket -= 1; System.out.println("姓名"+name); System.out.println("剩余票数:"+ticket); }else{ System.out.println("姓名"+name); System.out.println("票数不足"); System.out.println("剩余票数"+ticket); } } } public static void main(String[] args){ MyTherad at = new MyTherad(); Thread t1 = new Thread(at); Thread t2 = new Thread(at); Thread t3 = new Thread(at); Thread t4 = new Thread(at); Thread t5 = new Thread(at); t1.setName("蒋桥"); t2.setName("刘毅"); t3.setName("李莉"); t4.setName("张海华"); t5.setName("王晓东"); t1.start(); t2.start(); t3.start(); t4.start(); t5.start(); }*/ public int tickets = 4; public synchronized void getTicket(String name) { if (tickets > 0) { try { Thread.sleep(1); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } System.out.println(name + "买走了第" + tickets-- + "张票"); } else { System.out.println("票没了"); } } public static void main(String[] args) { MyTherad mt = new MyTherad(); Thread t1 = new Thread(mt); t1.setName("甲"); Thread t2 = new Thread(mt); t2.setName("乙"); Thread t3 = new Thread(mt); t3.setName("丙"); Thread t4 = new Thread(mt); t4.setName("丁"); Thread t5 = new Thread(mt); t5.setName("二"); t1.start(); t2.start(); t3.start(); t4.start(); t5.start(); } } class MyTherad implements Runnable{ public Text3 bank = new Text3(); @Override public void run() { (bank).getTicket(Thread.currentThread().getName()); } }
写一个程序统计一个项目中src下的所有 .java 文件的代码行数(用流的知识)
import java.io.BufferedReader; import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.io.Reader; //写一个程序统计一个项目中src下的所有 .java 文件的代码行数(用流的知识) public class Text2 { private static int daima; private static int kongbai; private static int zhushi; public static void main(String[] args){ File file = new File("F:\java\lianxi\0717\Text1\src\com\hanqi\Data.java"); if (file.exists()) { countor(file); System.out.println("代码行数: " + daima); System.out.println("空白行数: " + kongbai); System.out.println("注释行数: " + zhushi); } else { System.out.println("找不到路径 !"); } } public static void countor(File file) { File[] f_list = file.listFiles(); for (File f : f_list) { if (f.isDirectory()) { countor(f); } else { try { boolean flag = false; Reader reader = new FileReader(f); BufferedReader br = new BufferedReader(reader); String s = null; while ((s = br.readLine()) != null) { System.out.println(s); if (s.trim().startsWith("/*")) { flag = true; } else if (s.trim().endsWith("*/")) { flag = false; } else if (flag) { zhushi++; } else if (s.trim().startsWith("https://")) { zhushi++; } else if (s.trim().length() != 0 && !(s.trim().startsWith("/*") && s.trim().startsWith("https://"))) { daima++; } else if (!flag&&s.trim().length() == 0) { kongbai++; } } br.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } } } }
版权声明:
本文来源网络,所有图片文章版权属于原作者,如有侵权,联系删除。
本文网址:https://www.bianchenghao6.com/h6javajc/2196.html